Understanding Hash Tables: Explanation, Applications, and Java’s HashMap
Hash tables are a fundamental data structure in computer science, providing efficient data retrieval and storage. In this blog post, we will explore what hash tables are, their applications, how to implement them using Java’s HashMap, and tackle a classic example problem: the two-sum problem.
What is a Hash Table?
A hash table is a data structure that uses a hash function to map keys to values, allowing for fast data retrieval. The primary operations—insert, delete, and search—can typically be performed in constant time, O(1), under ideal circumstances.
How Hash Tables Work
- Hash Function: A hash function takes an input (the key) and produces a fixed-size string of characters, which typically appears random. This string is used as an index in the table.
- Collision Resolution: When two keys hash to the same index, a collision occurs. Hash tables handle collisions using techniques like chaining (linking entries at the same index) or open addressing (finding another open slot).
Applications of Hash Tables
Hash tables are widely used due to their efficiency and versatility. Some common applications include:
- Database Indexing: Quick lookups in large datasets.
- Caches: Storing frequently accessed data for fast retrieval.
- Counting Frequencies: Keeping track of occurrences of items (like words in a document).
- Sets: Implementing collections that do not allow duplicate entries.
Implementing Hash Tables with Java’s HashMap
Java provides a built-in class called HashMap
, which implements the hash table data structure. It allows for the storage of key-value pairs, where keys are unique.
Basic Operations
Here’s a quick overview of how to use HashMap
in Java:
javaCopy codeimport java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// Creating a HashMap
HashMap<String, Integer> map = new HashMap<>();
// Inserting values
map.put("Apple", 1);
map.put("Banana", 2);
map.put("Orange", 3);
// Retrieving values
System.out.println("Apple: " + map.get("Apple"));
// Checking existence
if (map.containsKey("Banana")) {
System.out.println("Banana exists in the map.");
}
// Removing values
map.remove("Orange");
// Iterating through the HashMap
for (String key : map.keySet()) {
System.out.println(key + ": " + map.get(key));
}
}
}
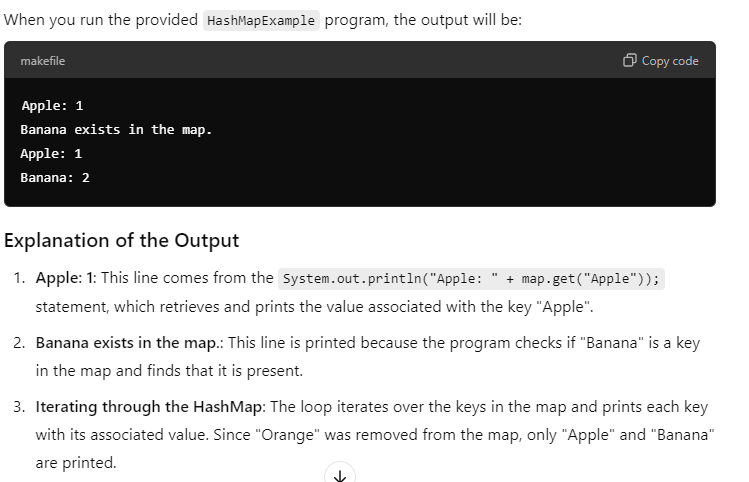
Key Features of HashMap
- Allows null values: You can have null as a key or value.
- Non-synchronized: It is not thread-safe, which means it is not suitable for concurrent access.
- Order: It does not maintain any order of elements; if you need order, consider
LinkedHashMap
.
Example Problem: The Two-Sum Problem
The two-sum problem is a classic interview question: Given an array of integers and a target sum, find two numbers that add up to that sum. Using a hash table, we can solve this efficiently.
Problem Statement
Given an array nums
and an integer target
, return the indices of the two numbers such that they add up to target
.
Solution Using HashMap
javaCopy codeimport java.util.HashMap;
public class TwoSum {
public static int[] twoSum(int[] nums, int target) {
HashMap<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
public static void main(String[] args) {
int[] nums = {2, 7, 11, 15};
int target = 9;
int[] result = twoSum(nums, target);
System.out.println("Indices: " + result[0] + ", " + result[1]);
}
}
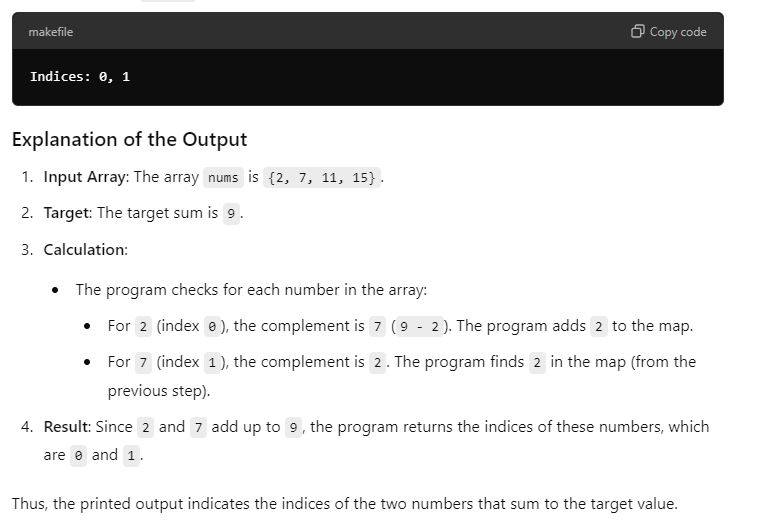
Explanation of the Solution
- Initialize a HashMap: This will store the value and its index as we iterate through the array.
- Loop through the array: For each number, calculate its complement (i.e.,
target - nums[i]
). - Check for the complement: If it’s in the map, return the indices. If not, add the current number and its index to the map.
Conclusion
Hash tables are a powerful data structure that enables efficient data retrieval and manipulation. Java’s HashMap
provides a straightforward way to implement this structure. By understanding hash tables and practicing problems like the two-sum problem, you can greatly enhance your programming skills.
Further Reading
- Explore more data structures and algorithms to deepen your understanding.
- Check out Java’s
ConcurrentHashMap
for thread-safe operations. - Practice more coding challenges on platforms like LeetCode and HackerRank.
By mastering hash tables, you’ll be better equipped to handle various coding challenges and improve the performance of your applications. Happy coding!
Also see: The Z Blogs
my other Blog: The Z Blog ZB